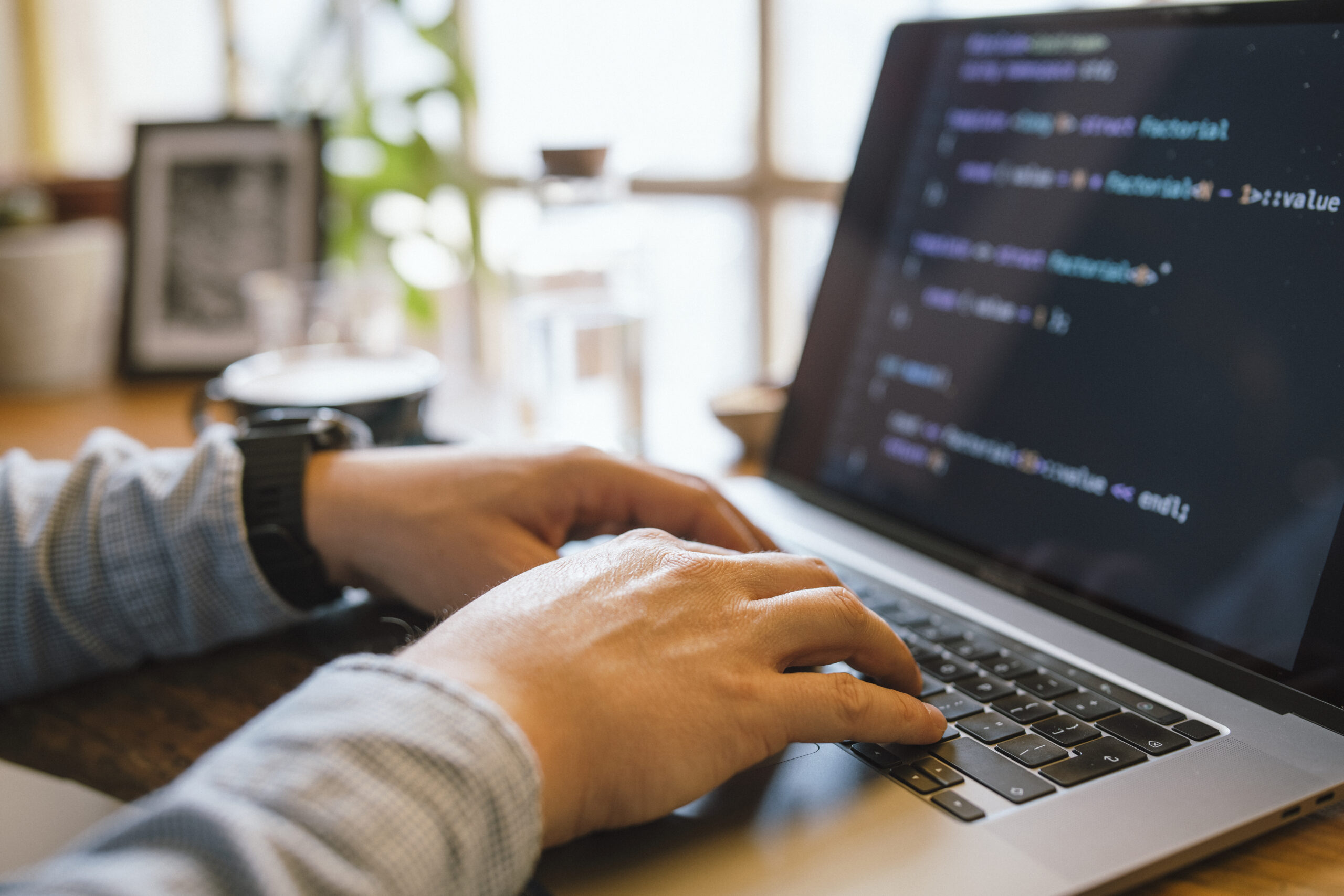
Debugging is One of the more crucial — still normally ignored — techniques inside of a developer’s toolkit. It's not pretty much fixing broken code; it’s about comprehending how and why items go Mistaken, and Mastering to Believe methodically to solve issues effectively. No matter whether you're a newbie or maybe a seasoned developer, sharpening your debugging techniques can help you save several hours of annoyance and considerably transform your efficiency. Here i will discuss quite a few procedures that will help builders stage up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest ways builders can elevate their debugging capabilities is by mastering the applications they use on a daily basis. Even though creating code is 1 Element of progress, knowing ways to communicate with it successfully during execution is Similarly crucial. Present day improvement environments occur Outfitted with effective debugging capabilities — but quite a few developers only scratch the surface area of what these applications can do.
Take, one example is, an Integrated Enhancement Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools help you set breakpoints, inspect the worth of variables at runtime, step through code line by line, and in many cases modify code within the fly. When made use of appropriately, they let you observe accurately how your code behaves through execution, which can be a must have for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, watch network requests, check out serious-time efficiency metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can turn annoying UI challenges into manageable duties.
For backend or system-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB provide deep Management around managing procedures and memory administration. Understanding these applications may have a steeper Understanding curve but pays off when debugging effectiveness concerns, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into comfortable with version Handle programs like Git to know code historical past, uncover the precise instant bugs were being released, and isolate problematic changes.
In the long run, mastering your instruments usually means likely beyond default settings and shortcuts — it’s about developing an intimate familiarity with your progress natural environment to make sure that when problems arise, you’re not lost at midnight. The higher you already know your instruments, the greater time it is possible to commit fixing the actual dilemma as an alternative to fumbling by way of the method.
Reproduce the trouble
Just about the most vital — and sometimes disregarded — measures in efficient debugging is reproducing the issue. Before leaping in the code or earning guesses, builders want to create a constant environment or state of affairs the place the bug reliably seems. With no reproducibility, fixing a bug becomes a activity of possibility, usually leading to wasted time and fragile code variations.
Step one in reproducing a problem is accumulating as much context as possible. Check with queries like: What actions led to The problem? Which surroundings was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The greater depth you've, the a lot easier it will become to isolate the exact disorders beneath which the bug occurs.
As you’ve collected more than enough data, try to recreate the challenge in your local setting. This might necessarily mean inputting the identical details, simulating equivalent person interactions, or mimicking method states. If The problem appears intermittently, look at writing automated assessments that replicate the sting circumstances or point out transitions associated. These assessments not only aid expose the problem but in addition protect against regressions in the future.
At times, the issue could be natural environment-specific — it might come about only on sure operating techniques, browsers, or less than specific configurations. Employing instruments like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these types of bugs.
Reproducing the trouble isn’t merely a move — it’s a state of mind. It needs persistence, observation, plus a methodical tactic. But when you can constantly recreate the bug, you happen to be by now midway to correcting it. Which has a reproducible state of affairs, You can utilize your debugging equipment far more properly, take a look at probable fixes safely and securely, and converse far more Evidently with your workforce or users. It turns an summary criticism right into a concrete problem — and that’s the place developers thrive.
Study and Comprehend the Error Messages
Mistake messages in many cases are the most worthy clues a developer has when a little something goes Completely wrong. Rather then looking at them as discouraging interruptions, developers ought to learn to treat mistake messages as immediate communications from your method. They often show you just what exactly occurred, the place it occurred, and sometimes even why it transpired — if you understand how to interpret them.
Begin by reading the concept very carefully and in full. Quite a few developers, specially when beneath time stress, look at the primary line and quickly begin earning assumptions. But further within the mistake stack or logs could lie the true root lead to. Don’t just copy and paste mistake messages into serps — go through and have an understanding of them 1st.
Crack the error down into pieces. Could it be a syntax error, a runtime exception, or maybe a logic error? Will it point to a certain file and line number? What module or operate brought on it? These issues can guidebook your investigation and issue you toward the dependable code.
It’s also helpful to grasp the terminology of your programming language or framework you’re making use of. Error messages in languages like Python, JavaScript, or Java usually abide by predictable designs, and Mastering to recognize these can dramatically hasten your debugging procedure.
Some problems are vague or generic, and in People cases, it’s vital to look at the context wherein the error occurred. Look at related log entries, input values, and recent improvements in the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger sized troubles and supply hints about opportunity bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Finding out to interpret them effectively turns chaos into clarity, encouraging you pinpoint troubles speedier, reduce debugging time, and become a more effective and assured developer.
Use Logging Properly
Logging is The most strong tools inside a developer’s debugging toolkit. When utilized efficiently, it provides genuine-time insights into how an application behaves, assisting you comprehend what’s happening beneath the hood with no need to pause execution or stage with the code line by line.
A great logging technique starts with knowing what to log and at what amount. Popular logging ranges include DEBUG, INFO, Alert, Mistake, and FATAL. Use DEBUG for comprehensive diagnostic info during enhancement, INFO for general situations (like thriving start out-ups), WARN for potential issues that don’t crack the appliance, ERROR for actual complications, and Lethal once the system can’t go on.
Prevent flooding your logs with extreme or irrelevant data. An excessive amount of logging can obscure important messages and decelerate your program. Focus on critical activities, state improvements, input/output values, and critical conclusion factors in your code.
Structure your log messages clearly and continually. Contain context, which include timestamps, request IDs, and function names, so it’s simpler to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what situations are met, and what branches of logic are executed—all devoid of halting the program. They’re Specially valuable in creation environments where stepping by way of code isn’t probable.
Furthermore, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about equilibrium and clarity. Using a very well-thought-out logging strategy, you could decrease the time it will require to identify problems, achieve further visibility into your applications, and improve the Total maintainability and trustworthiness of your code.
Consider Like a Detective
Debugging is not merely a specialized process—it is a form of investigation. To efficiently establish and take care of bugs, builders ought to approach the process just like a detective fixing a secret. This mindset assists break down intricate problems into manageable elements and observe clues logically to uncover the foundation cause.
Begin by gathering evidence. Look at the signs of the problem: mistake messages, incorrect output, or performance problems. Much like a detective surveys a crime scene, gather as much related info as you are able to without having leaping to conclusions. Use logs, take a look at scenarios, and consumer studies to piece collectively a clear image of what’s happening.
Subsequent, form hypotheses. Request oneself: What might be creating this behavior? Have any variations a short while ago been designed on the codebase? Has this concern occurred just before below similar instances? The target is always to narrow down possibilities and establish likely culprits.
Then, check your theories systematically. Try to recreate the issue inside of a managed atmosphere. If you suspect a certain perform or ingredient, isolate it and confirm if the issue persists. Just like a detective conducting interviews, inquire your code queries and Enable the final results lead you nearer to the truth.
Fork out close notice to tiny details. Bugs generally cover in the minimum expected spots—like a lacking semicolon, an off-by-one mistake, or a race issue. Be thorough and affected individual, resisting the urge to patch The problem website without the need of completely being familiar with it. Short term fixes may perhaps conceal the true problem, only for it to resurface afterwards.
And finally, continue to keep notes on Everything you tried out and learned. Just as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future challenges and assist Some others realize your reasoning.
By wondering like a detective, developers can sharpen their analytical capabilities, solution issues methodically, and turn into more practical at uncovering concealed issues in sophisticated programs.
Create Exams
Producing checks is among the most effective methods to increase your debugging techniques and In general development efficiency. Assessments not simply enable capture bugs early but will also function a security Web that offers you confidence when making modifications in your codebase. A effectively-examined application is simpler to debug since it permits you to pinpoint just the place and when a difficulty happens.
Begin with unit tests, which target specific features or modules. These modest, isolated assessments can swiftly reveal regardless of whether a particular piece of logic is working as envisioned. Every time a take a look at fails, you straight away know wherever to glance, drastically minimizing the time spent debugging. Device assessments are Specifically helpful for catching regression bugs—issues that reappear just after previously remaining fastened.
Following, integrate integration checks and conclude-to-stop tests into your workflow. These assistance be certain that different parts of your software get the job done collectively smoothly. They’re significantly valuable for catching bugs that happen in elaborate programs with numerous factors or companies interacting. If something breaks, your assessments can inform you which A part of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to definitely think critically regarding your code. To test a element correctly, you would like to comprehend its inputs, expected outputs, and edge scenarios. This degree of knowledge Normally sales opportunities to better code construction and much less bugs.
When debugging an issue, composing a failing test that reproduces the bug might be a robust initial step. As soon as the test fails persistently, you can give attention to correcting the bug and watch your examination go when The difficulty is resolved. This technique makes certain that exactly the same bug doesn’t return Down the road.
In short, creating assessments turns debugging from the disheartening guessing sport into a structured and predictable course of action—encouraging you catch much more bugs, more rapidly plus much more reliably.
Take Breaks
When debugging a difficult challenge, it’s easy to become immersed in the issue—watching your display screen for hrs, hoping Alternative after Answer. But Just about the most underrated debugging equipment is actually stepping absent. Having breaks allows you reset your mind, reduce aggravation, and often see the issue from a new standpoint.
If you're much too near the code for much too extensive, cognitive exhaustion sets in. You may begin overlooking obvious errors or misreading code that you wrote just several hours before. With this condition, your brain turns into much less effective at problem-resolving. A brief wander, a coffee break, or even switching to another undertaking for ten–15 minutes can refresh your focus. Lots of builders report obtaining the root of a problem when they've taken time and energy to disconnect, allowing their subconscious operate inside the background.
Breaks also assistance protect against burnout, Specially in the course of longer debugging classes. Sitting before a display screen, mentally stuck, is don't just unproductive but in addition draining. Stepping away allows you to return with renewed Electrical power plus a clearer state of mind. You may perhaps out of the blue discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
In case you’re stuck, a fantastic rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then have a 5–ten minute split. Use that point to move all-around, stretch, or do a thing unrelated to code. It might sense counterintuitive, Specifically less than tight deadlines, but it surely in fact leads to more rapidly and more practical debugging Over time.
To put it briefly, taking breaks is just not an indication of weakness—it’s a wise system. It provides your brain House to breathe, improves your viewpoint, and allows you steer clear of the tunnel eyesight that often blocks your progress. Debugging is often a psychological puzzle, and rest is a component of fixing it.
Master From Every Bug
Every single bug you come upon is more than just A brief setback—It can be a possibility to develop for a developer. Whether it’s a syntax error, a logic flaw, or possibly a deep architectural challenge, every one can teach you some thing useful when you go to the trouble to replicate and analyze what went Incorrect.
Commence by asking by yourself some vital concerns after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with superior techniques like device screening, code testimonials, or logging? The solutions typically expose blind spots within your workflow or knowing and allow you to Create much better coding patterns transferring ahead.
Documenting bugs can be a fantastic routine. Preserve a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring challenges or prevalent problems—you could proactively stay clear of.
In staff environments, sharing Whatever you've realized from a bug with your friends could be Particularly powerful. Irrespective of whether it’s via a Slack message, a brief publish-up, or a quick knowledge-sharing session, serving to Other folks avoid the exact same difficulty boosts staff efficiency and cultivates a much better Finding out culture.
Extra importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As opposed to dreading bugs, you’ll get started appreciating them as vital elements of your progress journey. In any case, some of the ideal developers will not be those who write ideal code, but individuals that continually master from their blunders.
Eventually, Every bug you deal with adds a whole new layer towards your skill established. So future time you squash a bug, take a minute to replicate—you’ll come away a smarter, extra capable developer as a result of it.
Conclusion
Strengthening your debugging competencies will take time, exercise, and tolerance — however the payoff is big. It will make you a more effective, self-confident, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s an opportunity to become far better at Anything you do.